TikTok Live API With Node.js
Intro
NOTICE: Make sure you understand TikTok’s community guidelines before proceeding.
TikTok has a lot of advanced features for a social media app. One thing that TikTok lacks is an API for handling chat interactions. For example, Twitch streamers will create events based on viewer interactions. This article will cover retrieving data from TikTok live streams. To do so we will be using Node.js and TikTok-Live-Connector.
Prerequisites:
- Node.js installed.
- Some JavaScript knowledge.
- Understanding of APIs (application programming interface).
- A TikTok live stream to analyze.
Setting up the project
To start, create a directory and initialize it as an npm project.
npm init
Next, install the Node package TikTok-Live-Connector. This allows the connection to TikTok live streams.
npm i tiktok-live-connector
Getting our first interactions
Next, start writing the program to connect to a TikTok live stream. The goal will be to connect to a TikTok live stream and retrieve data from the stream.
Setup initial application
First, create our application file at the default location ./index.js
.
In the application file, add the TikTok-Live-Connector package.
// Include TikTok-Live-Connector package.
const { WebcastPushConnection } = require('tiktok-live-connector');
Now we can write a program to connect to a TikTok live stream.
Setup connection to watch a specific TikTok Account
This example establishes a TikTok account to watch. Then it creates a connection with the TikTok-Live-Connector package. At this point, the application will either establish a connection, or fail.
// A TikTok account to watch. The account has to be live.
const username = 'pizzamannickdiesslin';
// Create a new connection.
const tiktokConnection = new WebcastPushConnection(username);
// Connect to the chat with our new object.
tiktokConnection.connect().then(state => {
console.info(`Connected to ${state.roomId}'s live`);
}).catch(err => {
console.error('Failed to connect', err);
})
Add a script to retrieve data
Next, add a script to retrieve data from the TikTok live stream.
This example will log information to the console every time someone comments.
// Listen for messages from the TikTok live stream.
tiktokConnection.on('chat', data => {
console.log(`User nickname: ${data.nickname}, Commented: ${data.comment}`);
})
Combining the script
The following is the final result of the script.
The script will do the following:
- Connect to TikTok live stream.
- Log each chat message to the Node.js console.
// Include TikTok-Live-Connector package.
const { WebcastPushConnection } = require('tiktok-live-connector');
// A TikTok account to watch. The account has to be live.
const username = 'pizzamannickdiesslin';
// Create a new connection.
const tiktokConnection = new WebcastPushConnection(username);
// Connect to the chat with our new object.
tiktokConnection.connect().then(state => {
console.info(`Connected to ${state.roomId}'s live`);
}).catch(err => {
console.error('Failed to connect', err);
})
// Listen for messages from the TikTok live stream.
tiktokConnection.on('chat', data => {
console.log(`User nickname: ${data.nickname}, Commented: ${data.comment}`);
/*
Example of the log:
User nickname: GreatPerson101, Commented: I love this stream!
*/
})
Running the script
To run the final script, run this command in the application directory. Assuming the application file is index.js.
node index.js
This program can get the data from the TikTok live stream. The next step would be planning what data to get from a live stream; then planning what to do with the data. It’s up to the programmer’s choice at that point, but there are a lot of possibilities!
TikTok-Live-Connector overview
The TikTok-Live-Connector library allows scripts to watch for many different stream interactions. This allows for many creative possibilities. Some ideas may be OBS interactions or controlling smart devices.
Here is a list of the TikTok events. It makes an API for TikTok live streams.
- member
- chat
- gift
- roomUser
- like
- social
- emote
- envelope
- questionNew
- linkMicBattle
- linkMicArmies
- liveIntro
- subscribe
Conclusion/Key Takeaways
In this example, we learned how to set up a Node.js application and retrieve data from a TikTok live stream.
3 takeaways:
- Even though TikTok doesn’t have an API we can retrieve live stream data.
- Node.js allows us to get creative with interactions. For example, we could run commands if people in the chat say certain things.
- TikTok-Live-Connector has many different commands to watch during TikTok live streams. This wraps TikTok’s website as an API.
TikTok currently doesn’t have an official API. The TikTok-Live-Connector allows developers to fetch data from live streams without an API. Be creative and have fun using this library. If you enjoy this library, please help support the maintainer. You also can help contribute to the repository on GitHub.
References
About the author
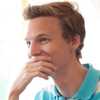
Written by Nicholas Diesslin Pizza acrobat 🍕, typographer, gardener, bicyclist, juggler, senior developer, web designer, all around whittler of the web.
Follow Nick on Twitter!